ArcadeX - Game ERP
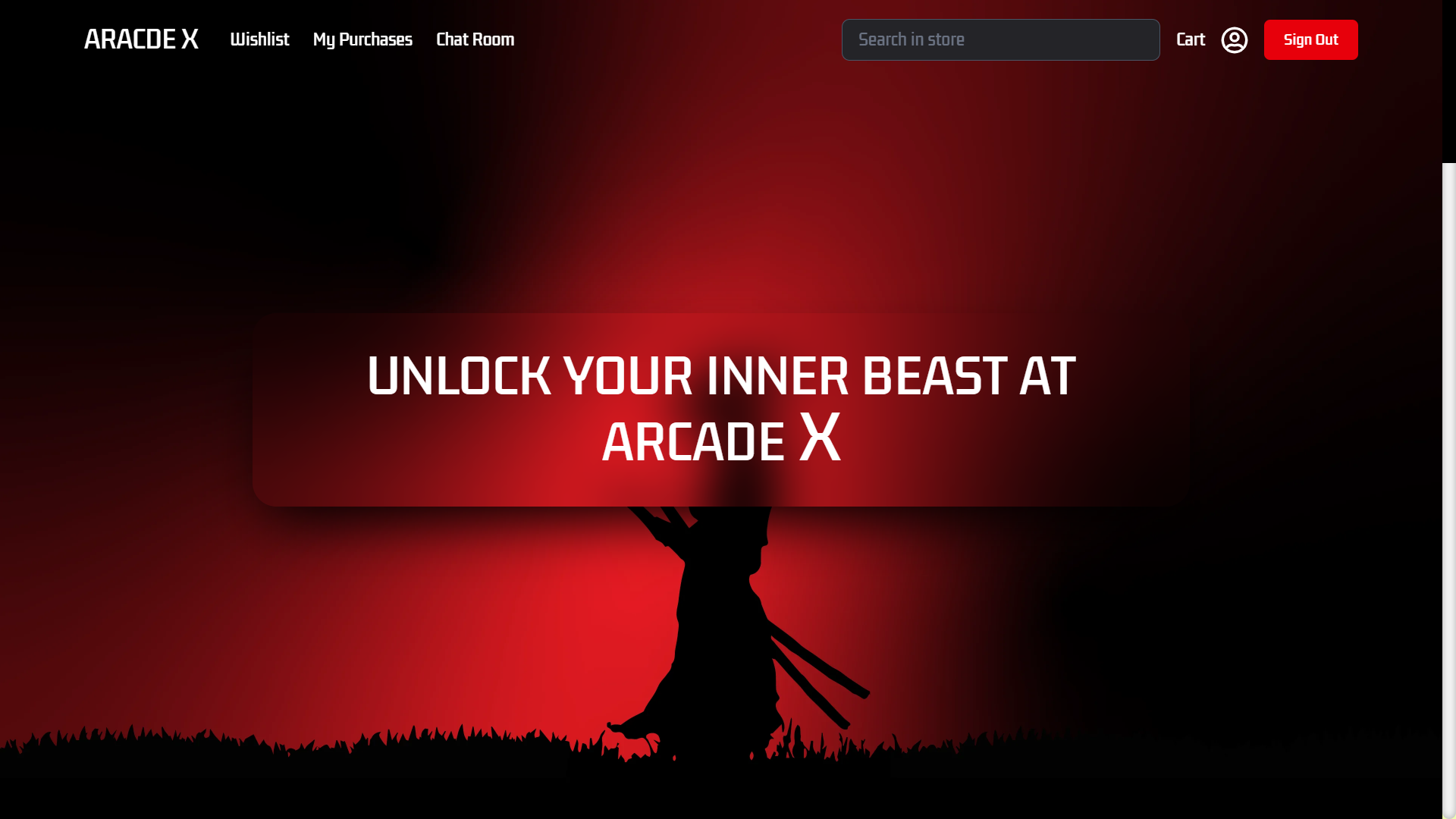
Project Overview
ArcadeX is a gaming marketplace where users can explore, purchase, and manage their favorite games. Built with a powerful backend and dynamic frontend, ArcadeX serves as an e-commerce platform for games. Sellers can upload their game listings, while administrators manage the platform, ensuring quality control and security.
App.jsx
1function App() {
2 const dispatch = useDispatch();
3 const { user, seller } = useSelector((store) => store);
4 const [loading, setLoading] = useState(true);
5
6 useEffect(() => {
7 const fetchUser = async () => {
8 try {
9 const responseGames = await axios.get(
10 "http://localhost:5000/api/game/landingPageGames",
11 { withCredentials: true }
12 );
13
14 dispatch(setGames(responseGames.data.data));
15
16 const type = localStorage.getItem("type");
17 const response = await axios.post(
18 "http://localhost:5000/api/auth/refresh",
19 { type: type },
20 { withCredentials: true }
21 );
22
23 if (response.data.length === 0) {
24 throw new Error("User not authenticated");
25 }
26
27 if (type === "User") {
28 dispatch(addUser(response.data.data));
29 } else if (type === "Seller") {
30 dispatch(addSeller(response.data.data));
31 }
32 } catch (error) {
33 console.error("Error fetching user:", error.message);
34 } finally {
35 setLoading(false);
36 }
37 };
38
39 if (!user && !seller) {
40 fetchUser();
41 } else {
42 setLoading(false);
43 }
44 }, [dispatch, user, seller]);
45
46 if (loading) {
47 return <LoadingScreen />;
48 }
49
50 //to see rest of the code, check github
Technology Stack
React
Javascript
Node.js
Express
MongoDB
Mongoose
Stripe Payment
Socket.io
Key Features
- Browse and explore a variety of games 📜
- Add games to cart and checkout via Stripe 💳
- Approve or reject games submitted by sellers
- Implemented role-based access control (RBAC) for users, sellers, and admins, including game approval workflows and user moderation, improving platform security
- Optimized database queries and API performance in a MERN stack architecture, reducing API response time by 40% and enhancing user experience.